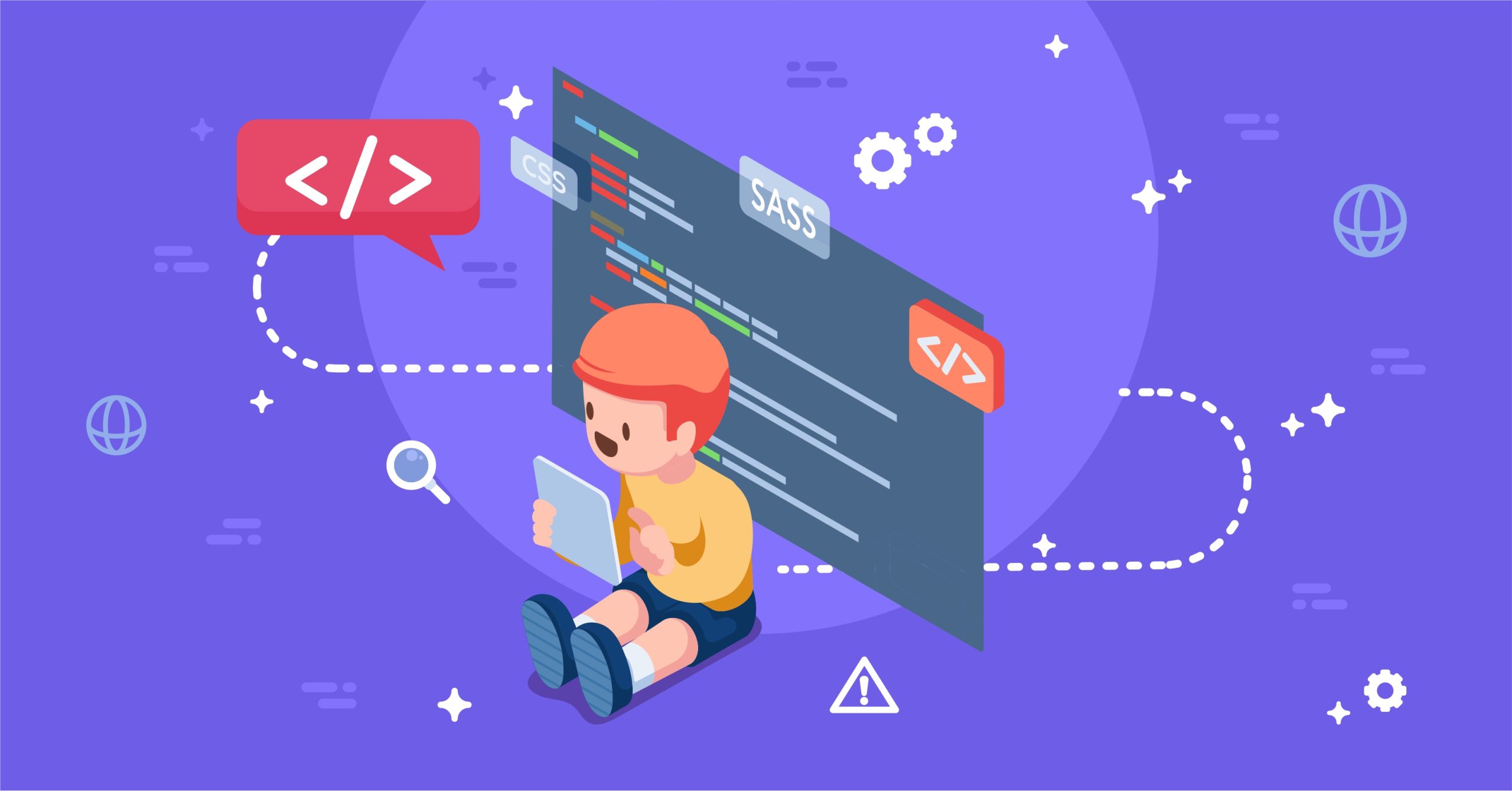
Web Development for Kids!: CSS & SASS
Web development is an exciting and creative activity, and it’s never too early to start! This guide is designed especially for young learners to introduce CSS and SASS, two crucial technologies for styling websites and making them look attractive and engaging. This is a follow-up from the web development for kids! Talking more about HTML in our previous article, if you haven’t gone through that, I suggest you go check it out, to have a wider knowledge of web development.
Importance of CSS and SASS in Web Development
CSS, which stands for Cascading Style Sheets, is a language used to describe how elements on a web page should look and be laid out. Whether you want to set a background color, change the font size, or arrange images in a neat grid, CSS is the tool for the job! It helps web developers create websites that are not only functional but also visually appealing and responsive to different devices like phones, tablets, and computers.
SASS, on the other hand, stands for Syntactically Awesome Stylesheets. It is a preprocessor scripting language that extends CSS by providing mechanisms such as variables, nested rules, and mixins, making CSS more powerful and easier to write. With SASS, you can write cleaner, more readable, and DRY (Don’t Repeat Yourself) code, which is especially beneficial as you work on larger projects.
What to Expect in This Article
This introductory article will pave the way for an adventurous journey into the world of web development for kids with a focus on CSS and SASS. You will learn:
- Basics of CSS: Understanding the fundamental principles and syntax of CSS.
- Introduction to SASS: An overview of SASS, how it differs from CSS, and why it’s beneficial for styling websites.
- Hands-on Examples & Exercises: Engage with practical examples and exercises designed for beginners to grasp the concepts better.
- Creative Projects: Towards the end, we will apply the learned concepts in building and styling a simple web page, providing a sense of accomplishment and a spark of creativity in young minds.
So, prepare for a fun and educational experience where you will acquire valuable skills that serve as a foundation for future learning in the dynamic field of web development!
Understanding CSS
What is CSS?
CSS, or Cascading Style Sheets, is a style sheet language used for describing the presentation of a document written in a markup language like HTML. Here’s a breakdown:
-
Definition: CSS controls the style and layout of web pages. It allows you to apply styles (like colors, fonts, and spacing) to web pages and their content.
-
Importance:
- Visual Appeal: It provides various styling options for creating visually engaging websites.
- Consistency: With CSS, you can ensure a consistent look and feel across various website pages.
- Responsive Design: CSS facilitates the development of web pages that are responsive to different devices and screen sizes.
Basic CSS Syntax
CSS is primarily made up of ‘selectors’ and ‘declaration blocks’:
- Selector: You pick which element or elements on the webpage to style.
- Declaration Block: This contains properties and values to apply styles to the selected element.
Here’s a basic syntax example:
selector {
property: value;
}
For instance:
body {
color: black;
background-color: white;
}
In this example, the body is the selector, color, and background-color are properties, and black and white are the respective values.
How to Include CSS in HTML
You can incorporate CSS into HTML in three ways:
**Inline Styles**
Inline styles are applied directly on individual HTML elements using the style attribute.
<p style="color:red;">This is a red paragraph.</p>
**Internal Styles**
Also known as embedded styles, these are placed within the head section of an HTML document using the style tag.
<!DOCTYPE html>
<html>
<head>
<style>
p {
color: blue;
}
</style>
</head>
<body>
<p>This is a blue paragraph.</p>
</body>
</html>
**External Style Sheets**
External styles are written in separate CSS files. You then link these files within the head section of your HTML document using the link element.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<p>This paragraph is styled with an external style sheet.</p>
</body>
</html>
And in the styles.css file:
p {
color: green;
}
For larger websites, external style sheets are recommended due to their efficiency and consistency in styling across multiple pages.
First Steps with CSS
Yay! Now that we know a little about CSS, let’s start having fun with it. In this section, we will play with colors, learn about the CSS box model, and understand how to place things where we want them on a web page.
Colors and Fonts
**Changing Text Color**
CSS lets you paint your text in any color you want! To set the color of text, use the `color` property.
Here's an example:
```css
p {
color: red;
}
This code will change the color of all paragraphs to red.
**Changing Font Style**
With CSS, you can change how the text looks by choosing different fonts using the font-family property.
Example:
p {
font-family: "Comic Sans MS", sans-serif;
}
Now, all paragraphs will appear in the Comic Sans MS font (or sans-serif if Comic Sans MS is not available).
Practice Exercise:
Try styling paragraphs with different colors and fonts! Write a few paragraphs in HTML and give them various colors and font styles with CSS. Experiment and see what you like best!
Box Model
What's the Box Model?
Every element on a web page is a box. The CSS box model has margins, borders, padding, and content. It’s like a series of nesting dolls!
Here's a simple explanation:
Content: This is where your text, images, or other content live.
Padding: The space between your content and the border.
Border: A line that goes around the padding and content.
Margin: The space between the border and the next element.
Leave a Reply