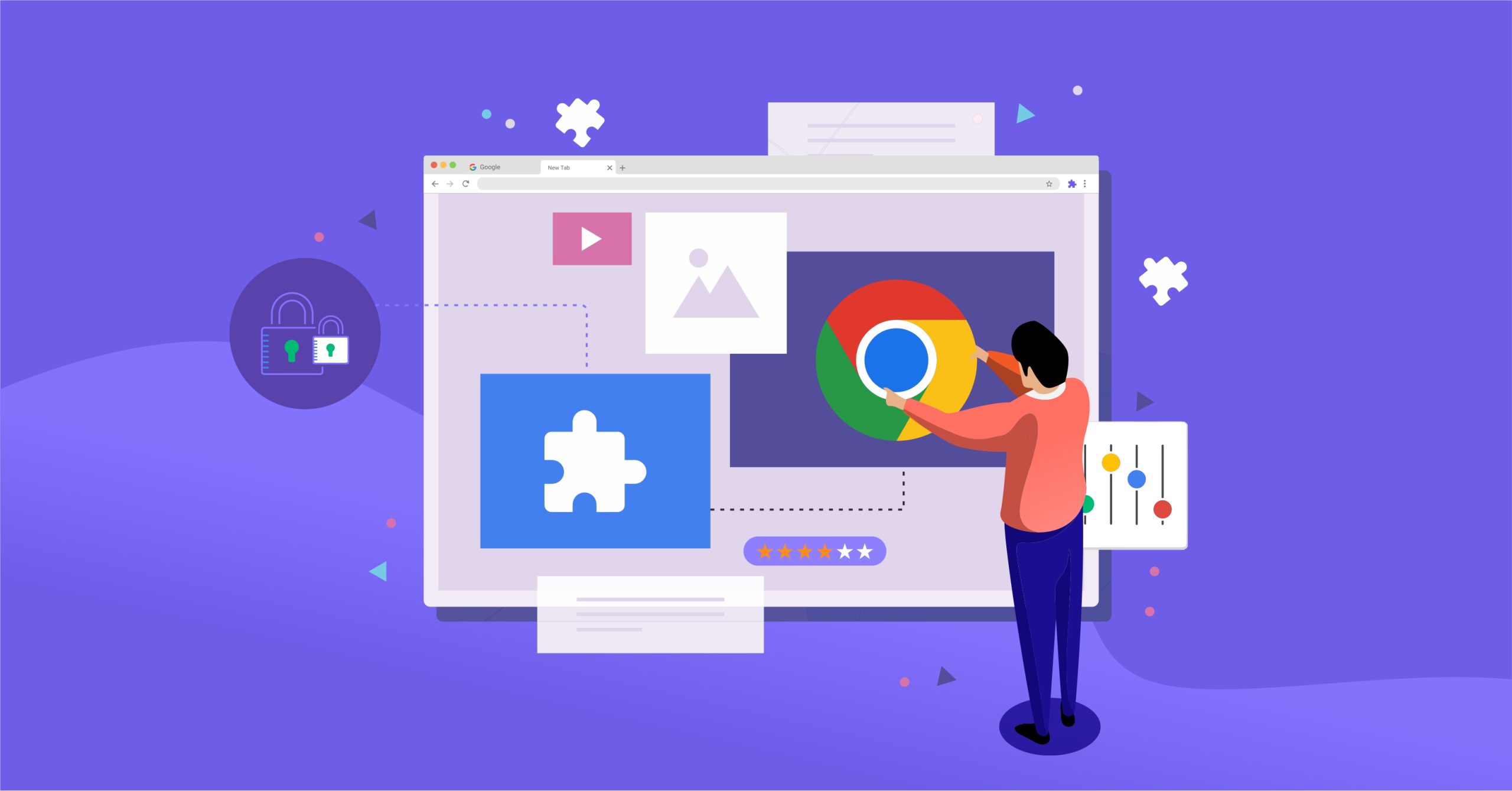
Create Your First Chrome Extension: A Simple Guide
Introduction
Chrome extensions are powerful tools that can enhance your browsing experience by adding custom functionalities to the Chrome browser. This guide aims to provide a comprehensive, step-by-step walkthrough for beginners to create their first Chrome extension. Before we dive into the details, let’s gain a brief understanding of what Chrome extensions are, their significance in personalizing browsing experiences, and the purpose of this guide.
Chrome Extensions: A Brief Overview
What are Chrome Extensions? Chrome extensions are small software programs that users can install to customize and enhance their browsing experience on the Google Chrome browser. These extensions add new features or modify existing functionalities, allowing users to tailor their browsers to meet their needs.
Functionality of Chrome Extensions: Chrome extensions can range from simple tools that change the appearance of a webpage to complex applications that interact with web services and provide advanced functionalities. They can modify the browser’s behavior, add new buttons to the toolbar, or automate repetitive tasks.
Importance of Creating Custom Chrome Extensions
Personalized Browsing Experience: Creating custom Chrome extensions allows users to tailor their browsing experience to match their preferences and requirements. Whether simplifying repetitive tasks, blocking specific content, or adding quick access to favorite websites, custom extensions empower users to shape their digital environment.
Efficiency and Productivity: Developing your Chrome extensions allows you to streamline your workflow, boost productivity, and save time. Instead of relying on generic extensions, crafting a personalized solution ensures that the functionalities align precisely with your needs, optimizing your online activities.
By understanding the concept of Chrome extensions and recognizing the benefits of creating your own, you’ll be well-equipped to develop personalized extensions that enhance your browsing experience and boost your productivity.
Purpose of the Guide
The primary goal of this guide is to empower beginners with the knowledge and skills to create their first Chrome extension. Navigating through the steps outlined in this guide, you’ll gain hands-on experience in extension development, from setting up your development environment to publishing your creation on the Chrome Web Store. Whether you’re a coding enthusiast or a curious user looking to delve into the world of extension development, this guide is designed to be your go-to resource for a smooth and educational journey.
Now, let’s embark on this exciting exploration of creating your first Chrome extension and unlock the potential to transform your browsing experience.
Section 1: Understanding Chrome Extensions
What are Chrome Extensions?
Definition and Explanation: Chrome extensions are small software programs that users can install to customize and enhance their browsing experience on the Google Chrome browser. These extensions augment the browser’s functionality, allowing users to tailor their digital environment by adding features, modifying behaviors, or interacting with web services.
Examples of Popular Chrome Extensions and Their Functionalities:
- AdBlock: A widely-used extension that blocks intrusive website ads, providing a cleaner and more focused browsing experience.
- Grammarly: A writing assistant that checks and suggests improvements to grammar, spelling, and punctuation as users type in text fields across the web.
- LastPass: A password manager that securely stores and auto-fills login credentials, enhancing security and convenience.
Section 2: Getting Started
Setting Up Your Development Environment
Installing the Necessary Tools: You must set up your development environment to embark on your Chrome extension development journey. Here are the essential tools to install:
-
Visual Studio Code (VS Code): A lightweight and versatile code editor that supports various programming languages. You can download it here.
-
Chrome Browser: The browser in which your extensions will run. If you don’t have Chrome installed, you can download it here.
Overview of the Chrome Extension Architecture: Before diving into your first project, it’s crucial to understand the architecture of a Chrome extension. This includes:
-
Manifest File: The manifest file is a JSON file that contains essential information about your extension, such as its name, version, permissions, and more.
-
Background Script: This is a JavaScript file that runs in the background and manages the extension’s functionality, even when the extension’s popup or content scripts are not actively running.
Creating Your First Extension Project
Initializing a New Extension Project: Once your development environment is set up, it’s time to initialize a new Chrome extension project. Use the following steps:
- Open VS Code and create a new folder for your project.
- create a file named
manifest.json
inside the project folder. This file will serve as the manifest file for your extension.
Understanding the Basic Structure of a Chrome Extension: The basic structure of a Chrome extension includes:
-
manifest.json: This file contains metadata about the extension, including its name, version, permissions, and more.
-
Background Script (background.js or background.ts): The JavaScript or TypeScript file that runs in the background and manages the extension’s functionality.
These fundamental components set the foundation for your extension project. Understanding them is crucial as you build and customize your extension. In the following sections, we’ll delve deeper into the components of a Chrome extension and guide you through creating your first extension project.
Section 3: Building Your Extension
Manifest File
Explanation of the Manifest File:
The manifest file, manifest.json
, serves as the blueprint for your Chrome extension. It contains vital information that defines the extension’s properties and functionalities. Here’s an overview of key components:
- Name: The name of your extension.
- Version: The version number of your extension.
- Description: A brief description of what your extension does.
- Permissions: Declares the permissions your extension needs to function, such as accessing tabs or storage.
Here is a simple example of a manifest file:
{
"manifest_version": 2,
"name": "My First Extension",
"version": "1.0",
"description": "A simple Chrome extension",
"permissions": ["storage", "tabs"],
"browser_action": {
"default_icon": "icon.png",
"default_popup": "popup.html"
}
}
Popup Page
Creating a Simple Popup Page:
A popup page is a small overlay that appears when the extension icon is clicked. It provides a quick way to interact with your extension. To create a popup page: Create an HTML file, e.g., popup.html, within your project. Design the popup using HTML to include the elements and layout you desire. Customize the CSS styles in a separate file, e.g., popup.css, to enhance the appearance. If interactivity is needed, add JavaScript functionality to the popup using a script file, e.g., popup.js. Here’s a basic example structure of a popup HTML file:
<!DOCTYPE html> <html lang=“en”> <head> <meta charset=“UTF-8”> <meta name=“viewport” content=“width=device-width, initial-scale=1.0”> <link rel=“stylesheet” href=“popup.css”> <title>My Extension Popup</title> </head> <body> <h1>Hello, this is my extension!</h1> <!– Add your popup content here –> <script src=“popup.js”></script> </body> </html>
Background Script Introduction to Background Scripts: Background scripts are JavaScript files that run in the background, independently of the popup or content scripts. They manage the extension’s functionality, handle events, and maintain state. Key points include: They operate globally across the browser. They can listen for events and respond accordingly.
Implementing Basic Background Script Functionalities: Create a JavaScript file, e.g., background.js, for your background script. Define the functionalities you want the background script to handle. Example background script: // background.js chrome.runtime.onInstalled.addListener(function() { console.log(“Extension installed!”); });
chrome.browserAction.onClicked.addListener(function(tab) { console.log(“Extension icon clicked!”); // Add your logic here }); These foundational components set the stage for your Chrome extension. In the upcoming sections, we’ll delve deeper into refining these elements and expanding the capabilities of your extension.
Section 4: Testing and Debugging
Testing Your Extension Locally
Loading the Extension in the Chrome Browser for Local Testing: To ensure your Chrome extension works as intended, local testing is crucial. Follow these steps to load your extension locally:
- Open the Chrome browser.
- Navigate to
chrome://extensions/
or click on the three dots in the top-right corner, go to “More tools,” and select “Extensions.” - Enable “Developer mode” at the top-right of the page.
- Click on “Load unpacked” and select the folder where your extension project is located.
Your extension should now appear in the list of installed extensions. If successful, you’ll see your extension icon in the browser toolbar.
Debugging Common Issues and Errors: During the testing phase, you may encounter issues or errors. Here are common debugging strategies:
-
Inspect Popup/Page Elements: Use Chrome’s Developer Tools to inspect elements, view console logs, and identify issues within your popup/page.
-
Console Logging: Integrate console.log statements in your JavaScript code to output information and debug messages. These logs can be viewed in the Developer Tools console.
-
Background Script Debugging: Inspect the background script by navigating to the “Extensions” page, finding your extension, and clicking “Inspect views: background page.”
-
Check Permissions: Ensure your manifest file accurately declares the necessary permissions. Permissions errors can lead to functionality issues.
-
Reload the Extension: After changing your code, click the “Reload” button next to your extension on the “Extensions” page to apply the updates.
Testing your extension locally and addressing any identified issues ensures a smoother user experience when the extension is published.
In the following sections, we’ll explore additional testing techniques and guide you through the process of publishing your extension to the Chrome Web Store.
Section 5: Publishing Your Extension
Preparing Your Extension for Publishing
Finalizing Your Extension’s Functionality: Before publishing your Chrome extension, ensure it is feature-complete and functions as intended. Conduct thorough testing to catch any remaining bugs or issues. Consider gathering user feedback to make informed improvements.
Ensuring Compliance with Chrome Web Store Policies: To successfully publish your extension on the Chrome Web Store, adhere to Chrome’s policies. Common compliance considerations include:
- Permissions: Only request necessary permissions. Overly broad permissions may lead to rejection.
- Content Guidelines: Ensure your extension complies with content guidelines to prevent rejection or removal.
- Trademark and Copyright: Avoid trademark and copyright infringements using original content or obtaining proper permissions.
Uploading Your Extension to the Chrome Web Store
Step-by-Step Guide on Creating a Developer Account:
- Google Account: Ensure you have a Google Account. If not, create one here.
- Chrome Developer Dashboard: Go to the Chrome Developer Dashboard.
- Agree to Terms: Agree to the Chrome Web Store Developer Agreement.
- Set Up Payments (if applicable): If you plan to sell your extension, set up payments.
Publishing Your Extension:
- Navigate to the Dashboard: Click on the “+ Create a new item” button.
- Upload Extension: Upload your extension’s ZIP file.
- Complete Required Fields: Fill in essential details, including a detailed description, screenshots, and promotional images.
- Publishing Options:
- Public or Private: Choose whether your extension will be publicly available or limited to a specific audience.
- Pricing (if applicable): Set the pricing model (free or paid).
- Countries: Specify the countries where your extension will be available.
- Review and Publish: Review the information and click “Publish Changes” to submit your extension for review.
Post-Publishing Considerations:
- Regularly update your extension to fix bugs and add new features.
- Engage with user reviews and feedback.
- Monitor for policy violations and address any issues promptly.
Congratulations! Your Chrome extension is now published on the Chrome Web Store. Users can discover, install, and benefit from your creation. Continue to enhance your extension based on user feedback and evolving requirements.
Conclusion
Recap of the Key Steps in Creating Your First Chrome Extension
In this guide, we embarked on a journey to create your first Chrome extension, covering various aspects of extension development. Let’s recap the key steps you’ve taken:
-
Understanding Chrome Extensions:
- Grasped the concept of Chrome extensions and their functionalities.
- Explored examples of popular extensions and their diverse uses.
-
Getting Started:
- Set up your development environment by installing Visual Studio Code and the Chrome browser.
- Gained an overview of the Chrome extension architecture.
-
Building Your Extension:
- Explored the critical components, starting with the manifest file.
- Created a simple popup page for your extension.
- Introduced background scripts and implemented basic functionalities.
-
Testing and Debugging:
- Learned how to test your extension locally by loading it into the Chrome browser.
- Explored debugging strategies to address common issues and errors.
-
Publishing Your Extension:
- Finalize your extension’s functionality.
- Ensured compliance with Chrome Web Store policies.
- Published your extension on the Chrome Web Store with a step-by-step guide.
Encouragement for Readers to Explore and Customize Their Extensions Further
Congratulations on reaching this point in your Chrome extension development journey! The world of Chrome extensions is vast and offers endless possibilities for customization. As you move forward, consider the following:
-
Continuous Learning: Stay updated on new features, best practices, and emerging technologies related to Chrome extension development.
-
User Feedback: Engage with users, respond to feedback, and use it to enhance your extension. Understanding user needs is critical to creating a successful extension.
-
Experimentation: Don’t be afraid to experiment with new functionalities and design elements. Customizing your extension based on your evolving preferences is part of the fun.
Remember, creating Chrome extensions is a dynamic and rewarding experience. Whether you’re developing for personal use or considering broader audiences, the skills you’ve acquired can open doors to a world of creative possibilities. Keep exploring, keep building, and enjoy the journey of crafting extensions that make your browsing experience uniquely yours.
Additional Resources
Links to Official Chrome Extension Documentation
Explore the official Chrome Extension documentation for in-depth information, guides, and references to advance your knowledge and skills in extension development.
Recommended Books, Tutorials, and Online Courses for Advanced Chrome Extension Development
-
Books:
- “Mastering Chrome Developer Tools” by Ryoichi Tsunekawa
- “Building Scalable Apps with Google Chrome” by Marc Rochkind
-
Tutorials:
-
Online Courses:
These resources offer advanced insights, hands-on tutorials, and in-depth learning materials to elevate your skills in Chrome extension development.
FAQs
Answers to Common Questions and Troubleshooting Tips for Beginners in Chrome Extension Development
Q: How do I debug my Chrome extension?
A: Use Chrome Developer Tools to inspect elements, view console logs, and debug JavaScript. You can also use the background script’s dedicated DevTools.
Q: Why is my extension not working after loading it locally?
A: Check the console for error messages in Chrome Developer Tools. Ensure the manifest file, permissions, and scripts are correctly configured.
Q: Can I update my extension after publishing it on the Chrome Web Store?
A: You can update your extension by uploading a new ZIP file with the updated code and version number on the Chrome Developer Dashboard.
Q: How can I handle cross-origin requests in my extension?
A: Declare the necessary permissions in the manifest file and use the permissions
key to specify the domains your extension needs access to.
Q: What are common reasons for extension rejection on the Chrome Web Store?
A: Common reasons include violating content guidelines, requesting unnecessary permissions, and infringing on trademarks or copyrights.
Refer to the official Chrome Extension documentation and community forums for more detailed troubleshooting and answers to specific questions.
Leave a Reply