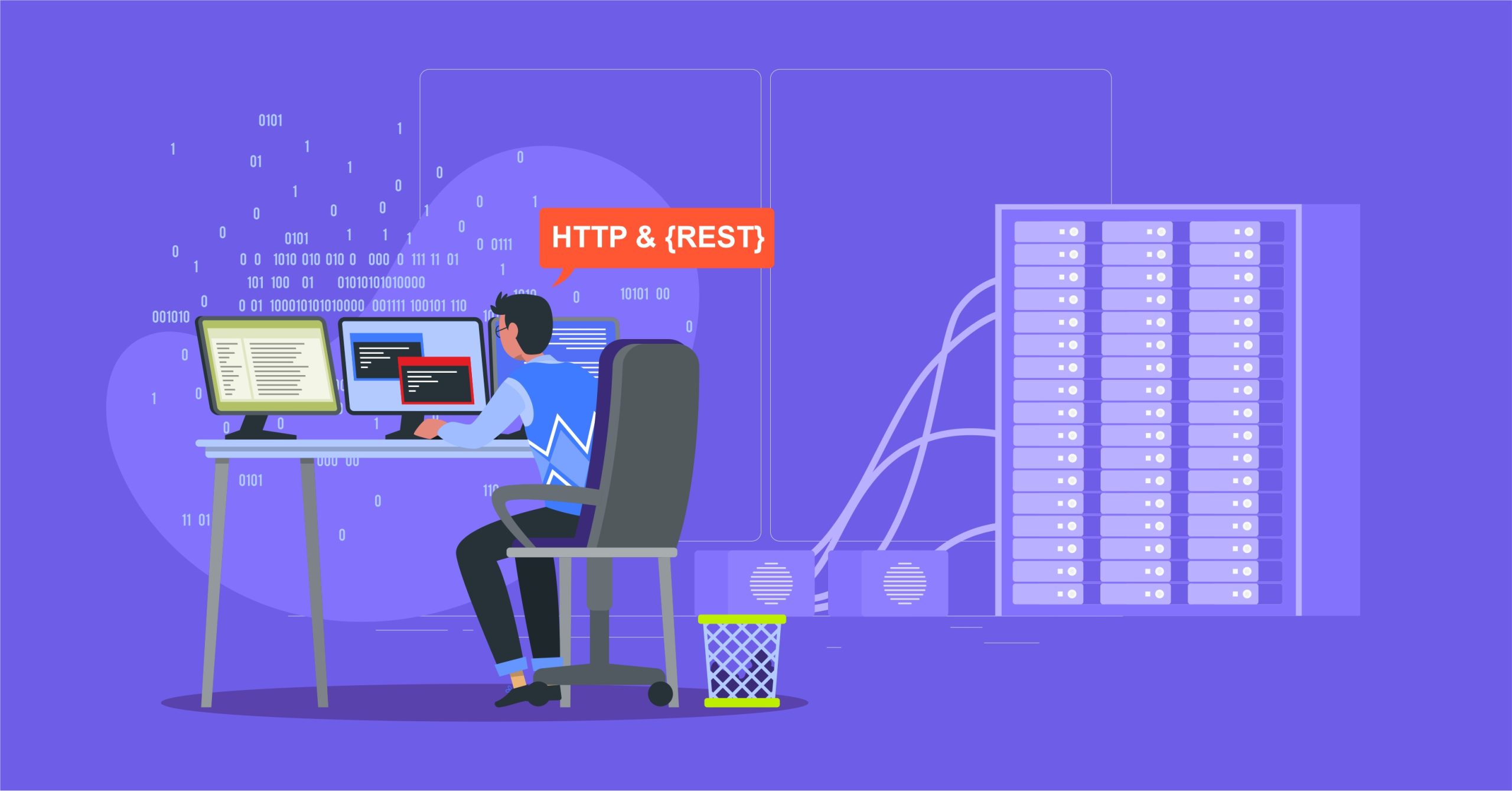
Understanding HTTP & REST
Introduction
In the dynamic landscape of web development, two fundamental technologies are pivotal in enabling seamless communication between clients and servers: HTTP (Hypertext Transfer Protocol) and REST (Representational State Transfer). This introduction briefly overviews their significance and sets the stage for comprehensively exploring their fundamentals.
Importance of HTTP and REST
HTTP: At the core of every web interaction, HTTP is the foundation for data communication. It governs how data is transmitted over the web, forming the backbone of the World Wide Web. Understanding HTTP is essential for developers as it facilitates the exchange of information between clients, such as browsers and servers, where web content resides.
REST: Representational State Transfer, or REST, is a set of architectural principles that guide the design of networked applications. RESTful APIs (Application Programming Interfaces) have become the standard for developing web services, providing a scalable and flexible approach to building modern applications. Mastery of REST principles empowers developers to create robust and efficient systems.
Enabling Communication
HTTP acts as the messenger between clients and servers, defining how requests and responses should be formatted. REST, built on top of HTTP, outlines a set of conventions for structuring these interactions. They facilitate data exchange, allowing applications to communicate, retrieve resources, and perform actions seamlessly.
Purpose of the Article
This article aims to delve deep into the fundamentals of HTTP and REST. From understanding the basics of HTTP methods to exploring the principles of RESTful architecture, this journey will equip readers with the knowledge needed to navigate the intricacies of web development. By the end, readers will grasp the technical aspects and appreciate the crucial role HTTP and REST play in shaping the modern digital experience.
Section 1: HTTP Basics
What is HTTP?
Definition and Explanation: HTTP, or Hypertext Transfer Protocol, is the foundation of data communication on the World Wide Web. It is an application layer protocol that transfers hypermedia, such as text, images, and videos, between clients (typically web browsers) and servers. HTTP forms the basis for any data exchange on the internet.
Client-Server Communication Model: HTTP operates on a client-server communication model. In this model, clients (devices or applications) make requests to servers, and servers respond to these requests. This interaction enables the retrieval and transmission of web resources, providing a standardized way for browsers to communicate with web servers.
Request-Response Cycle: The request-response cycle is a fundamental concept in HTTP. When a client sends a request to a server, it specifies the action it wants to perform (e.g., fetching a web page). The server processes the request and returns a response containing the requested data or an indication of the success or failure of the operation. This cycle is the basis of all interactions on the web.
HTTP Methods
Introduction to HTTP Methods: HTTP methods, also known as verbs, define the type of operation the client requests to be performed on a resource. The most common methods include:
- GET: Retrieve data.
- POST: Submit data.
- PUT: Update a resource.
- DELETE: Remove a resource.
Purpose and Use Cases: Each HTTP method serves a specific purpose. GET is used for retrieving data, POST for submitting data, PUT for updating resources, and DELETE for removing resources. Understanding when to use each method is crucial for building practical and secure web applications.
Examples of HTTP Methods:
- A GET request may retrieve information from a server.
- A POST request can submit a form on a web page.
- A PUT request might update user information.
- A DELETE request could remove a specific record from a database.
HTTP Headers
Explanation of HTTP Headers: HTTP headers are additional information sent with a request or response that provides metadata about the communication. They are crucial in controlling and describing how messages should be handled.
Commonly Used Headers:
- Content-Type: Specifies the type of data in the body of the request or response (e.g., JSON or HTML).
- Accept: Informs the server about the data types the client can handle.
- User-Agent: Identifies the software and version making the request, usually the browser or user agent.
Status Codes
Overview of HTTP Status Codes: HTTP status codes are three-digit numbers that provide information about the status of a request. They indicate whether the request was successful, encountered an error, or needs further action.
Different Status Code Categories:
- 1xx (Informational): Indicates that the request was received and is continuing the process.
- 2xx (Successful): Indicates that the request was successfully received, understood, and accepted.
- 3xx (Redirection): Indicates further action needs to be taken to complete the request.
- 4xx (Client Error): Indicates that the client seems to have made an error.
- 5xx (Server Error): Indicates that the server failed to fulfill a valid request.
Examples of Common Status Codes:
- 200 OK: Request was successful.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: A generic error message returned when an unexpected condition was encountered.
This foundational understanding of HTTP basics forms the basis for effective web development and lays the groundwork for exploring more advanced concepts in subsequent sections.
Section 2: REST Principles
What is REST?
Definition and Explanation: REST, or Representational State Transfer, is an architectural style that defines principles for designing networked applications. It was introduced by Roy Fielding in his doctoral dissertation in 2000. RESTful systems are characterized by their simplicity, scalability, and statelessness.
Key Principles of RESTful Architecture:
- Statelessness: Each request from a client to a server must contain all the information needed to understand and fulfill the request. The server should not store information about the client’s state between requests.
- Client-Server Architecture: The client and server are separate entities communicating over a stateless protocol (usually HTTP). This separation allows for independent development and scalability of each component.
- Uniform Interface: The interface between clients and servers should be uniform to simplify and decouple the architecture. This principle is realized through the use of standard conventions and constraints.
Introduction to Resources, URIs, and Statelessness:
- Resources: In REST, everything is a resource. Resources can represent real-world entities like users, products, or abstract ideas.
- URIs (Uniform Resource Identifiers): Resources are uniquely identified using URIs, providing a standard way to access and manipulate them.
- Statelessness: The statelessness of REST means that each request is independent, and the server does not store any client state. This simplifies the architecture and improves scalability.
RESTful APIs
Explanation of RESTful APIs: A RESTful API is an interface that adheres to the principles of REST. It allows clients to interact with server resources using standard HTTP methods. RESTful APIs are widely used in web development for building scalable and maintainable systems.
Key Characteristics of RESTful APIs:
- Resource-Based: Resources are the fundamental abstractions in RESTful APIs, and a unique URI identifies each resource.
- CRUD Operations: RESTful APIs use standard HTTP methods for CRUD operations—Create (POST), Read (GET), Update (PUT/PATCH), and Delete (DELETE).
- Representation: Resources may have different representations, such as JSON or XML, depending on client requirements.
Examples of Real-World RESTful API Implementations:
- GitHub API: Provides access to various GitHub resources, allowing developers to interact with repositories, issues, and users.
- Twitter API: Allows developers to integrate Twitter functionality into their applications, enabling actions like posting tweets and retrieving user data.
RESTful CRUD Operations
Explanation of CRUD Operations in the Context of REST:
- Create (POST): Used to create a new resource on the server.
- Read (GET): Used to retrieve a representation of a resource from the server.
- Update (PUT/PATCH): Used to modify an existing resource on the server.
- Delete (DELETE): Used to remove a resource from the server.
RESTful Best Practices
Overview of Best Practices for Designing RESTful APIs:
- Versioning: Implement versioning to handle changes to the API without disrupting existing clients.
- Authentication: Use secure authentication mechanisms to protect resource access.
- Error Handling: Provide clear and informative error messages to assist developers in troubleshooting.
- HATEOAS (Hypermedia As The Engine Of Application State): Include hypermedia links in responses to guide clients through the application.
These RESTful principles and best practices lay the groundwork for designing scalable, maintainable, and interoperable web systems. Understanding and applying these concepts is essential for developers working with modern web architectures.
Section 3: Practical Applications
Case Studies
Analysis of Well-Known Applications or Services:
-
GitHub:
- Overview: GitHub, a version control and collaborative software development platform, extensively uses RESTful APIs.
- Adherence to REST Principles: GitHub’s API follows RESTful principles by treating resources (repositories, issues, users) as entities with unique URIs and employing standard HTTP methods for CRUD operations.
-
Twitter:
- Overview: Twitter’s API allows developers to interact with Twitter’s services programmatically.
- Adherence to REST Principles: Twitter’s API follows RESTful architecture, with resources identified by URIs and CRUD operations performed using standard HTTP methods.
-
Salesforce:
- Overview: Salesforce, a cloud-based customer relationship management (CRM) platform, provides a REST API for integration with its services.
- Adherence to REST Principles: Salesforce’s RESTful API follows the principles of statelessness, resource identification through URIs, and standard HTTP methods.
- Consistent Resource Naming: Analyzing how applications maintain consistency in naming resources through URIs.
- Use of Standard HTTP Methods: Evaluating how CRUD operations are implemented using standard HTTP methods.
- Statelessness: Assessing whether applications adhere to the statelessness principle, ensuring that each request contains the necessary information for the server to fulfill it.
Tools and Libraries
Introduction to Tools and Libraries for Working with HTTP and REST:
-
Postman:
- Overview: Postman is a popular API development and testing tool that simplifies the process of building and testing APIs.
- Usage: Developers can create requests, inspect responses, and automate API testing using Postman’s user-friendly interface.
-
Axios:
- Overview: Axios is a JavaScript library for making HTTP requests from browsers or Node.js applications.
- Usage: Axios simplifies making asynchronous HTTP requests and handling responses in JavaScript applications.
-
Express.js:
- Overview: Express.js is a web application framework for Node.js that simplifies the creation of APIs and web applications.
- Usage: Developers can quickly use Express.js to build RESTful APIs, taking advantage of its middleware and routing capabilities.
Practical Examples:
- Postman:
- Create a new request. - Specify the request method, URI, and any required headers. - Send the request and analyze the response, checking for status codes and data.
Axios: // Example of making a GET request using Axios axios.get(‘/api/resource’) .then(response => { console.log(response.data); }) .catch(error => { console.error(‘Error:’, error); });
Express.js: // Example of creating a simple RESTful API endpoint using Express.js const express = require(‘express’); const app = express();
app.get(‘/api/resource’, (req, res) => { res.json({ message: ‘Hello, this is a RESTful endpoint!’ }); });
app.listen(3000, () => { console.log(‘Server is running on port 3000’); });
These case studies and tools provide practical insights into how HTTP and REST are applied in real-world scenarios, and the tools mentioned simplify the development and testing processes for working with these technologies.
Section 4: Future Trends
HTTP/2 and Beyond
Overview of HTTP/2 and Its Improvements:
-
Introduction to HTTP/2:
- HTTP/2 is the second major version of the HTTP network protocol which the World Wide Web uses. It was developed to address the limitations of HTTP/1.1 and improve the efficiency of data transfer between clients and servers.
-
Key Improvements Over HTTP/1.1:
- Multiplexing: Enables multiple requests and responses to be sent concurrently over a single connection, reducing latency.
- Header Compression: Reduces overhead by compressing HTTP headers, optimizing data transmission.
- Stream Prioritization: Allows prioritization of specific streams, enhancing the overall performance of web applications.
-
** Emerging Protocols:**
- HTTP/3 is the latest version of the Hypertext Transfer Protocol that aims to optimize performance using the QUIC transport protocol. It focuses on reducing latency and enhancing security, especially in scenarios with unreliable network conditions.
GraphQL
Introduction to GraphQL and Its Role in API Development:
-
Definition of GraphQL:
- GraphQL is a query language for APIs and a runtime environment for executing those queries with the existing data. It provides a more efficient, powerful, and flexible alternative to traditional REST APIs.
-
Key Characteristics:
-
Declarative Data Fetching: Clients can request only the data they need, minimizing over-fetching or under-fetching of data.
-
Single Endpoint: GraphQL APIs typically expose a single endpoint, simplifying the communication process between clients and servers.
-
Strong Typing: GraphQL APIs are strongly typed, ensuring consistency and predictability in data structures.
-
Flexibility: GraphQL allows clients to request specific data, avoiding over-fetching or multiple requests for related data.
-
Single Endpoint: Unlike REST, which often involves multiple endpoints, GraphQL typically relies on a single endpoint for all data queries.
-
Versioning: GraphQL reduces the need for versioning as clients can specify the exact data they require.
-
These future trends in HTTP, with the evolution from HTTP/2 to HTTP/3, and the emergence of GraphQL, showcase the ongoing efforts to enhance the efficiency and flexibility of communication protocols and API development practices.
Conclusion
In conclusion, this exploration of HTTP and REST has provided a foundational understanding of these crucial technologies in web development. Let’s recap the key concepts learned:
HTTP Basics:
- Client-Server Communication: HTTP facilitates communication between clients and servers, enabling the transfer of hypertext and data.
- Request-Response Cycle: The interaction between clients and servers follows a request-response cycle, where clients send requests, and servers respond accordingly.
- HTTP Methods and Status Codes: HTTP methods (GET, POST, PUT, DELETE) are used for CRUD operations, and status codes convey the outcome of requests.
REST Principles:
- Resource-Based Architecture: RESTful systems treat entities as resources, each identified by a unique URI.
- Statelessness: REST adheres to the statelessness principle, ensuring that each request contains the necessary information for the server to fulfill.
- Uniform Interface: The uniform interface simplifies and decouples the architecture using standard conventions and constraints.
Practical Applications:
Case Studies:
- GitHub, Twitter, Salesforce: Examined how well-known applications adhere to REST principles, focusing on resource naming, HTTP methods, and statelessness.
Tools and Libraries:
- Postman, Axios, Express.js: Explored practical tools and libraries for working with HTTP and REST, simplifying API testing and development tasks.
Future Trends:
HTTP/2 and Beyond:
- Multiplexing, Header Compression, Stream Prioritization: Highlighted improvements in HTTP/2 over HTTP/1.1.
- HTTP/3: Discussed the emerging protocol HTTP/3 and its focus on reducing latency and enhancing security.
GraphQL:
- Declarative Data Fetching, Single Endpoint, Strong Typing: Introduced GraphQL and compared its characteristics with traditional RESTful APIs.
Further Exploration
As web development evolves, staying informed about advancements in communication protocols like HTTP and alternative approaches like GraphQL is essential. The journey doesn’t end here – further explore the practical applications of these technologies. Embrace the continuous learning mindset, experiment with tools, and contribute to the ever-growing field of web development.
Happy coding!
Additional Resources
Books
-
“HTTP: The Definitive Guide” by David Gourley and Brian Totty
-
“RESTful Web Services” by Leonard Richardson and Sam Ruby
Tutorials
-
MDN Web Docs – HTTP Overview
-
RESTful API Design – Best Practices in a Nutshell
Online Courses
-
Coursera – “Web Design and Development” by University of London
-
Udemy – “RESTful Web Services with Spring Boot” by in28Minutes Official
Official Documentation
-
MDN Web Docs – HTTP Protocol
-
REST API Documentation – OpenAPI Specification (OAS)
These resources provide a wealth of information for deepening your knowledge of HTTP and REST. Whether you prefer books, interactive tutorials, or structured courses, there’s something for every learning style. Explore the official documentation for comprehensive insights into the technical details of these technologies.
Leave a Reply