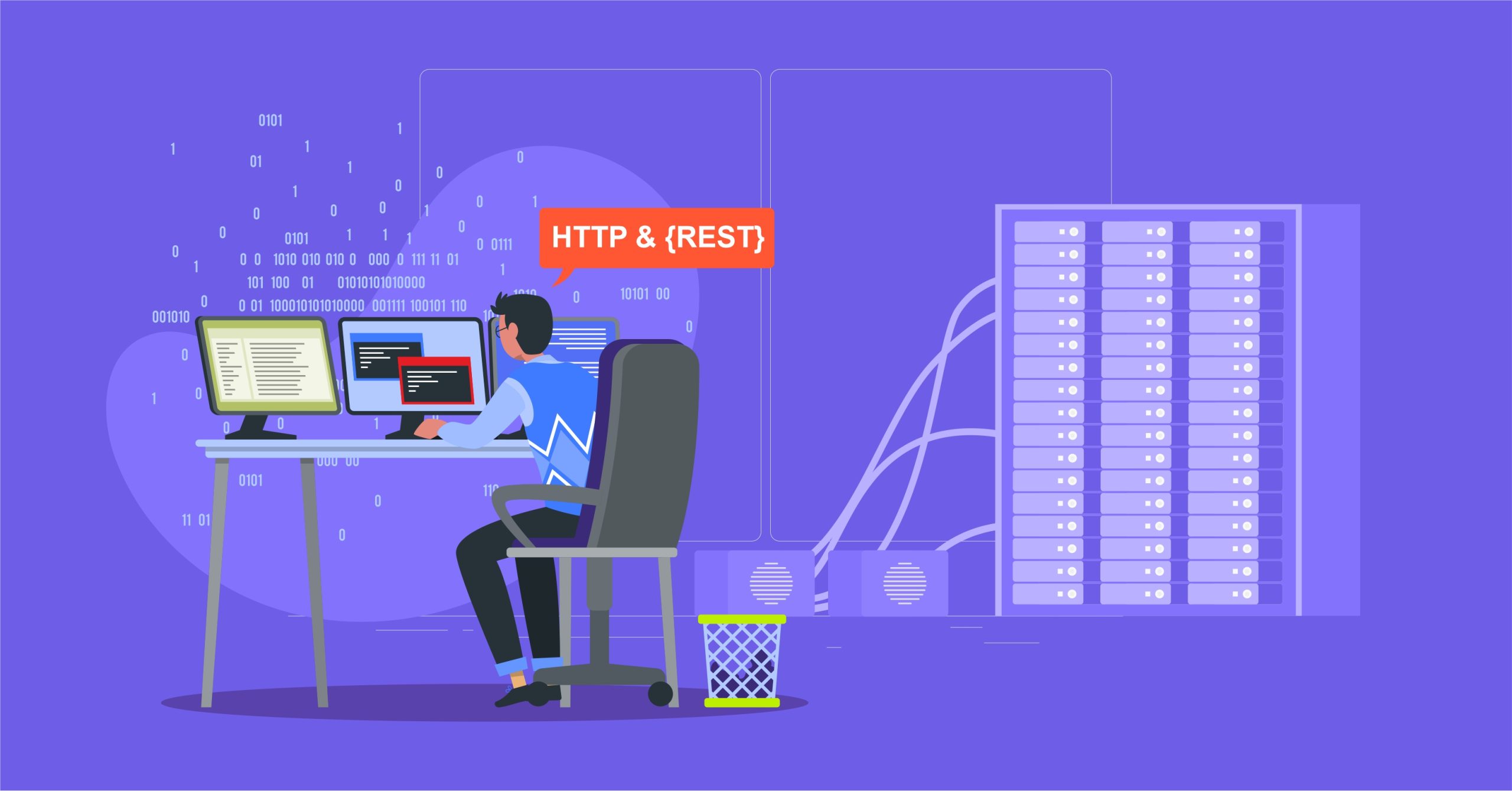
Understanding REST & HTTP: A Beginner’s Guide
In the digital age, the World Wide Web has become an integral part of our daily lives, and web applications have revolutionized how we interact with information and services. Whether ordering your favorite meal online, streaming your favorite TV show, or browsing the web, you’re likely interacting with a web application.
These applications rely on a foundational set of technologies and principles, and at the core of this ecosystem are HTTP and REST. In this beginner’s guide, we’ll explore the fundamentals of building web applications with HTTP and REST, including understanding HTTP and different HTTP request types, structuring REST APIs, and determining suitable response codes.
REST
REST, representing Representational State Transfer, is an architectural style and constraint for designing networked applications, mainly web services. It was introduced by Roy Fielding in his doctoral dissertation in 2000 and has since become a fundamental concept in web development.
RESTful web services are designed to be simple, scalable, and stateless, making them ideal for building APIs and interacting with online resources.
Now that you understand what REST is let’s explore HTTP in the next section.
HTTP
HTTP (Hypertext Transfer Protocol) is the backbone of the World Wide Web. The protocol enables communication between a client (typically a web browser) and a server. This communication is at the heart of how web applications work.
HTTP operates on a request-response model. Below is an oversimplified explanation of how it works:
-
Client Sends a Request: The client, which is usually a web browser, sends an HTTP request to a web server. This request includes various details, such as the requested resource’s URL, the request method, headers, and sometimes a request body (used for data transmission, e.g., when submitting a form).
-
Server Processes the Request: Upon receiving the request, the web server processes it. This may involve fetching data from a database, running some business logic, or performing other tasks based on the request.
-
Server Sends a Response: After processing the request, the server sends an HTTP response back to the client. This response includes an HTTP status code, headers, and a response body (usually
HTML
,JSON
, or other data).
The HTTP protocol has several methods or request types, allowing clients to specify the desired action on the server.
Let’s dive into these request types next.
Different HTTP Request Types
HTTP defines several request methods (also known as HTTP verbs) to indicate the desired action to be performed on the server. Each method has a specific purpose and meaning. The most commonly used request methods include:
-
GET
: TheGET
method requests data from the server. It’s considered a safe and idempotent operation, meaning it should not have any side effects on the server’s data. -
POST
: ThePOST
method is used to submit data to be processed by the identified resource. It is not idempotent and often results in data creation or modification on the server. -
PUT
: ThePUT
method is used to update a resource or create it if it doesn’t exist. It’s idempotent, meaning multiple requests with the same data will have the same effect. -
DELETE
: As the name suggests, theDELETE
method is used to request the removal of a resource from the server. It is idempotent if the resource is either deleted or not found. -
PATCH
: ThePATCH
method is used to apply partial modifications to a resource. It’s often used when you want to update only specific fields of a resource, making it more efficient thanPUT
in some cases. -
HEAD
: TheHEAD
method is similar toGET
but only requests the resource’s headers, not the actual data. Checking for resource existence and retrieving metadata without transferring the full content is useful. -
OPTIONS
: TheOPTIONS
method describes the target resource’s communication options. It’s often used to check the server’s allowed methods or other capabilities.
These request methods are integral to HTTP and play a significant role in building web applications.
Let’s look at how to use them effectively in your web development projects.
How to Use HTTP Request Methods
Understanding the purpose of each HTTP request method is just the first step. To effectively use these methods, you must know how to structure your requests and handle responses.
The following is a brief overview of how to use each method:
GET
The GET
method requests data from the server, typically retrieving web pages, images, or other resources. The request is typically sent as follows:
GET /resource-path
Host: www.example.com
- The path specifies the resource you want to retrieve.
- The
Host
header indicates the domain name of the server.
The server then responds with the requested resource, along with relevant headers and a status code.
POST
POST
requests are used to send data to the server, often for creating new resources or updating existing ones. A common use case is submitting a form on a web page. Here’s a basic example:
POST /create-resource
Host: example.com
Content-Type: application/x-www-form-urlencoded
key1=value1&key2=value2
- The
Content-Type
header indicates the data format being sent. - The request body contains the data to be processed by the server.
The server processes the data and responds with an appropriate status code and, if applicable, a response body.
PUT
PUT
requests are used to update an existing resource or create it if it doesn’t exist. Here’s how a PUT
request might look:
PUT /update-resource/123
Host: example.com
Content-Type: application/json
{
"key1": "new-value"
}
- The URL specifies the resource to be updated (in this case, resource with ID 123).
- The
Content-Type
header indicates the format of the request body.
The server processes the request and returns an appropriate status code and, if applicable, a response body.
DELETE
DELETE
requests are used to request the removal of a resource from the server. Here’s a simple example:
DELETE /remove-resource/456
Host: example.com
- The URL specifies the resource to be deleted (in this case, resource with ID 456).
The server processes the request, removes the resource, and responds with an appropriate status code.
PATCH
PATCH
requests are used to apply partial modifications to a resource. Here’s a basic example:
PATCH /update-resource/789
Host: example.com
Content-Type: application/json-patch+json
[
{ "op": "replace", "path": "/key1", "value": "new-value" }
]
- The URL specifies the resource to be updated (in this case, resource with ID 789).
- The
Content-Type
header indicates the format of the request body, which is typically in JSON Patch format.
The server processes the request, applies the specified modifications, and responds with an appropriate status code.
HEAD
HEAD
requests are similar to GET
requests but do not include the response body. They are used to retrieve metadata about a resource. Here’s an example:
HEAD /resource-info
Host: example.com
The server responds with headers and an appropriate status code but without the actual resource content.
OPTIONS
OPTIONS
requests are used to describe the communication options for the target resource. They are often used to check the allowed methods and other capabilities of the server. Here’s an example:
OPTIONS /resource-options
Host: example.com
The server responds with information about the allowed methods, headers, and other communication options.
Now that we understand the fundamentals of HTTP and the various request methods let’s explore how to structure RESTful APIs, which provide a systematic way to design and interact with web services.
Structuring REST APIs
RESTful APIs adhere to principles and constraints, which help create scalable and maintainable web services.
Here are some best practices for structuring REST APIs:
1. Resource-Based URLs
In a RESTful API, a unique URL should identify each resource (e.g., a user, product, or order). These URLs should be hierarchical and organized in a meaningful way. For example:
/users
to access a list of users./users/123
to access a specific user with the ID 123.
2. Use HTTP Methods
HTTP methods (GET
, POST
, PUT
, DELETE
, PATCH
, etc.) should be used to perform CRUD
(Create, Read, Update, Delete) operations on resources. The choice of method should be aligned with the action you want to perform.
GET /users
to retrieve a list of users.POST /users
to create a new user.PUT /users/123
to update user 123.DELETE /users/123
to delete user 123.
3. Statelessness
Each request from a client to the server must contain all the information needed to understand and process the request. The server should not store any client state. This means that the API should be designed so that it doesn’t rely on previously received requests.
4. Use of HTTP Status Codes
HTTP status codes are essential for conveying the result of an operation. Some common HTTP status codes include:
200 OK
: The request was successful.201 Created
: The request resulted in creating a new resource.204 No Content
: The request was successful, but there is no response body.400 Bad Request
: The request was malformed or invalid.404 Not Found
: The requested resource was not found.500 Internal Server Error
: An error occurred on the server.
Using appropriate status codes makes it easier for clients to understand the outcome of their requests.
5. HATEOAS (Hypermedia as the Engine of Application State)
This principle suggests that a RESTful API should provide links or references to related resources in the responses. Clients should be able to navigate the API by following these links, reducing the need for prior knowledge of the API’s structure.
6. Versioning
Including a version number in the API URL is common practice to ensure backward compatibility as the API evolves. For example:
/v1/users
for version 1 of theusers
resource./v2/users
for version 2 of theusers
resource.
By following these principles, you can design RESTful APIs that are easily understood, scalable, and interoperable. Now, let’s discuss the importance of HTTP status codes in RESTful API development.
Determining Suitable Response Codes
HTTP status codes are essential in communicating the outcome of an HTTP request. They provide information about whether the request was successful, encountered an error, or required further action. Understanding and choosing the right status codes is crucial for building effective RESTful APIs. Here are some common HTTP status codes and their meanings:
200 OK
The 200
status code indicates that the request was successful, and the server has returned the requested data. It’s commonly used for successful GET
requests.
201 Created
This status code is used when a new resource is successfully created due to a POST
request. The response typically includes information about the newly created resource, such as its location.
204 No Content
When a request is successful, but there is no response body to return (e.g., for a successful DELETE
request), the server can respond with a 204
status code.
400 Bad Request
The 400
status code is used when the server cannot understand or process the client’s request due to a client error. This may occur if the request is malformed or missing the required parameters.
401 Unauthorized
The 401
status code indicates that the request requires authentication and the client failed to provide valid credentials. The client should typically reauthenticate and retry the request.
403 Forbidden
A 403
status code means that the server understood the request, but it refuses to authorize it. This status is often used for situations where the client has insufficient permissions.
404 Not Found
When a requested resource does not exist on the server, the server responds with a 404
status code. This is one of the most recognizable status codes, often seen in web browsing.
500 Internal Server Error
The 500
status code indicates that the server encountered an error while processing the request. This error is not the result of a client mistake and should be investigated on the server side.
503 Service Unavailable
A 503
status code is used when the server is temporarily unable to handle the request due to maintenance or overloading. It indicates that the client should try the request again later.
Choosing the right status code is critical for accurately conveying a request’s outcome. It helps clients and developers understand the nature of the response and how to react to it. A well-designed RESTful API should consistently use appropriate status codes to ensure clarity and reliability.
Conclusion
In the realm of web development, understanding HTTP and REST is fundamental for building efficient and scalable web applications. HTTP is the protocol that powers the World Wide Web, and REST is the architectural style that helps structure web services. By using HTTP request methods effectively, following REST principles for API design, and choosing appropriate HTTP status codes, you can create web applications and services that are robust and reliable.
As a beginner, it’s essential to grasp the concepts of HTTP and REST, as they form the foundation upon which more advanced web development skills are built. As you continue your journey in web development, you’ll find that these principles remain constant and play a vital role in creating successful web applications that serve users and businesses alike.
Leave a Reply